#DS1307 #DS3231 #I2C
Ứng dụng: Lưu thời gian thực cho thiết bị, hẹn giờ bật tắt, lập lịch
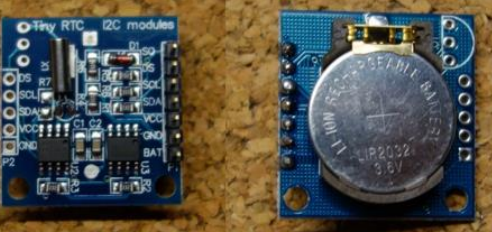
Như bạn có thể thấy trong hình trên, mô-đun đã lắp đặt pin. Điều này cho phép mô-đun giữ lại thời gian, ngay cả khi nó không được cung cấp nguồn bởi Arduino. Bằng cách này, mỗi khi bạn bật và tắt mô-đun của mình, thời gian sẽ được lưu giữ. Mô-đun này sử dụng giao tiếp I2C. Điều này có nghĩa là nó giao tiếp với Arduino chỉ sử dụng 2 chân.
Cách nối dây
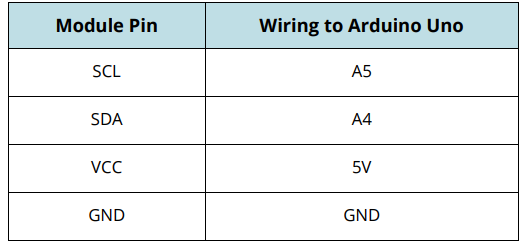
Nếu bạn sử dụng bo mạch Arduino khác chứ không phải Uno, hãy xem SCL và chân SDA. của nó dưới đây:
- Nano: SDA (A4); SCL(A5)
- MEGA: SDA (20); SCL(21)
- Leonardo: SDA (20); SCL(21)
Hiển thị ngày giờ ra Serial Monitor
Sơ đồ mạch
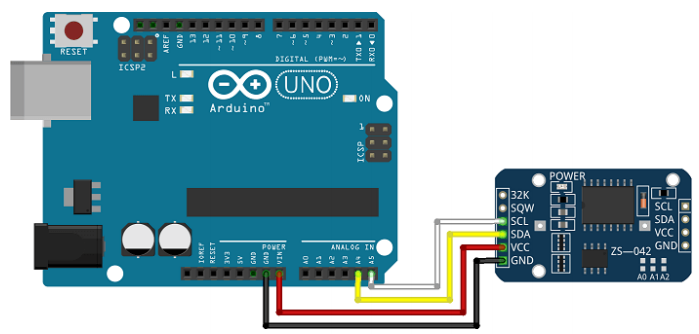
Chương trình
Làm việc với RTC đòi hỏi phải thực hiện hai bước quan trọng:
- Cài đặt thời gian hiện tại để RTC biết bây giờ là mấy giờ.
- Phải luôn có điện từ PIN hoặc từ nguồn ngoài để RTC lưu lại thời gian và đưa ra thời gian chính xác.
Đặt thời gian hiện tại trong Đồng hồ thời gian thực
Để thiết lập thời gian hiện tại, bạn cần đặt thời gian trong hàm setDS3231time()

Các tham số được tô sáng màu đỏ: giây, phút, giờ, ngày trong tuần, ngày, tháng và năm (theo thứ tự này). Chủ nhật thì ngày trong tuần là 1, đến thứ 7 là 6 (day = 6).
// Written by John Boxall from http://tronixstuff.com
#include "Wire.h"
#define DS3231_I2C_ADDRESS 0x68
// hàm chuyển số cơ số 10 sang BCD
byte decToBcd(byte val){
return( (val/10*16) + (val%10) );
}
// chuyển BCD sang hệ 10
byte bcdToDec(byte val){
return( (val/16*10) + (val%16) );
}
void setup(){
Wire.begin();
Serial.begin(9600);
// Khởi tạo thời gian ban đầu, thường khi lập trình, ta sẽ đồng độ DSxxx từ network hoặc qua giao diện cài giờ từ các nút nhấn:
// DS3231 seconds, minutes, hours, day, date, month, year
setDS3231time(30,42,16,5,13,10,16);
}
void setDS3231time(byte second, byte minute, byte hour, byte dayOfWeek, byte
dayOfMonth, byte month, byte year){
// cài giờ DS3231
Wire.beginTransmission(DS3231_I2C_ADDRESS);
Wire.write(0); // set next input to start at the seconds register
Wire.write(decToBcd(second)); // set seconds
Wire.write(decToBcd(minute)); // set minutes
Wire.write(decToBcd(hour)); // set hours
Wire.write(decToBcd(dayOfWeek)); // set day of week (1=Sunday, 7=Saturday)
Wire.write(decToBcd(dayOfMonth)); // set date (1 to 31)
Wire.write(decToBcd(month)); // set month
Wire.write(decToBcd(year)); // set year (0 to 99)
Wire.endTransmission();
}
void readDS3231time(byte *second,
byte *minute,
byte *hour,
byte *dayOfWeek,
byte *dayOfMonth,
byte *month,
byte *year){
Wire.beginTransmission(DS3231_I2C_ADDRESS);
Wire.write(0); // set DS3231 register pointer to 00h
Wire.endTransmission();
Wire.requestFrom(DS3231_I2C_ADDRESS, 7);
// request seven bytes of data from DS3231 starting from register 00h
*second = bcdToDec(Wire.read() & 0x7f);
*minute = bcdToDec(Wire.read());
*hour = bcdToDec(Wire.read() & 0x3f);
*dayOfWeek = bcdToDec(Wire.read());
*dayOfMonth = bcdToDec(Wire.read());
*month = bcdToDec(Wire.read());
*year = bcdToDec(Wire.read());
}
void displayTime(){
byte second, minute, hour, dayOfWeek, dayOfMonth, month, year;
// retrieve data from DS3231
readDS3231time(&second, &minute, &hour, &dayOfWeek, &dayOfMonth, &month,
&year);
// in ra Monitor Serial
Serial.print(hour, DEC);
// convert the byte variable to a decimal number when displayed
Serial.print(":");
if (minute<10){
Serial.print("0");
}
Serial.print(minute, DEC);
Serial.print(":");
if (second<10){
Serial.print("0");
}
Serial.print(second, DEC);
Serial.print(" ");
Serial.print(dayOfMonth, DEC);
Serial.print("/");
Serial.print(month, DEC);
Serial.print("/");
Serial.print(year, DEC);
Serial.print(" ngay trong Tuan: ");
switch(dayOfWeek){
case 1:
Serial.println("Chu Nhat");
break;
case 2:
Serial.println("Thu 2");
break;
case 3:
Serial.println("Thu 3");
break;
case 4:
Serial.println("Thu 4");
break;
case 5:
Serial.println("Thu 5");
break;
case 6:
Serial.println("Thu 6");
break;
case 7:
Serial.println("Thu 7");
break;
}
}
void loop(){
displayTime(); // hiện thị giờ
delay(1000); // sau 1s
}